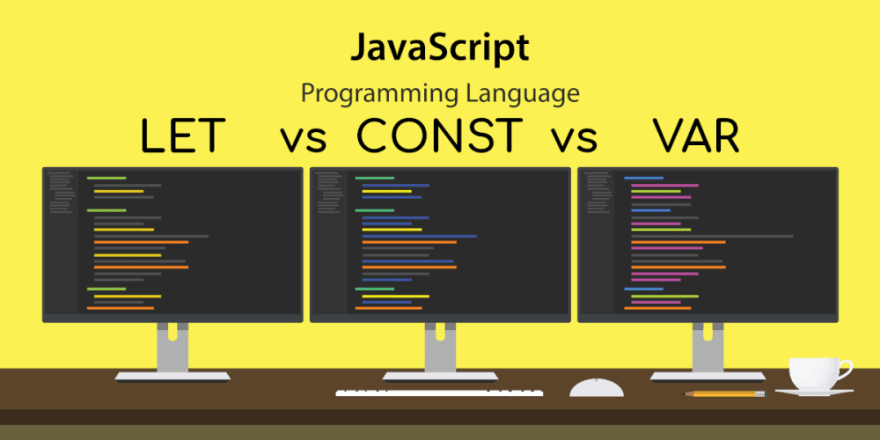
Difference Between Var, Let and Const
We have to know about scope before discuss about these three. Scope means where these variable can be used.
Var: var is the oldest method of variable declaration in JS. The declaration of Var are scoped globaly or locally. Global scope means when you declare var outside of a Function. By global scope makes you use that declared var anywhere in the window. On the other hand when you declare a var inside a function then that can only accesseble inside that function. These scope is known as local scope. Again var can be re-declared and updated. This is the main problem of var. This is why let and const come in.
Let: Let is now preferred for variable declaration. It actually comes as an improvement to var declaration. Let is block scoped. A block lives in curly braces. So, variable which is declared in a block with let can only be accessible within that block. When it comes redeclaration unlike var, let cannot be re-declareable. But you can update the value if you want.
Const: Variable declared with the const maintain constant values. Const declaration are similar to the let declaration. Like let declaration const are block scoped. That means const can only be accessible inside a block. But the main power of const is you cannot update or re-declared const varible.
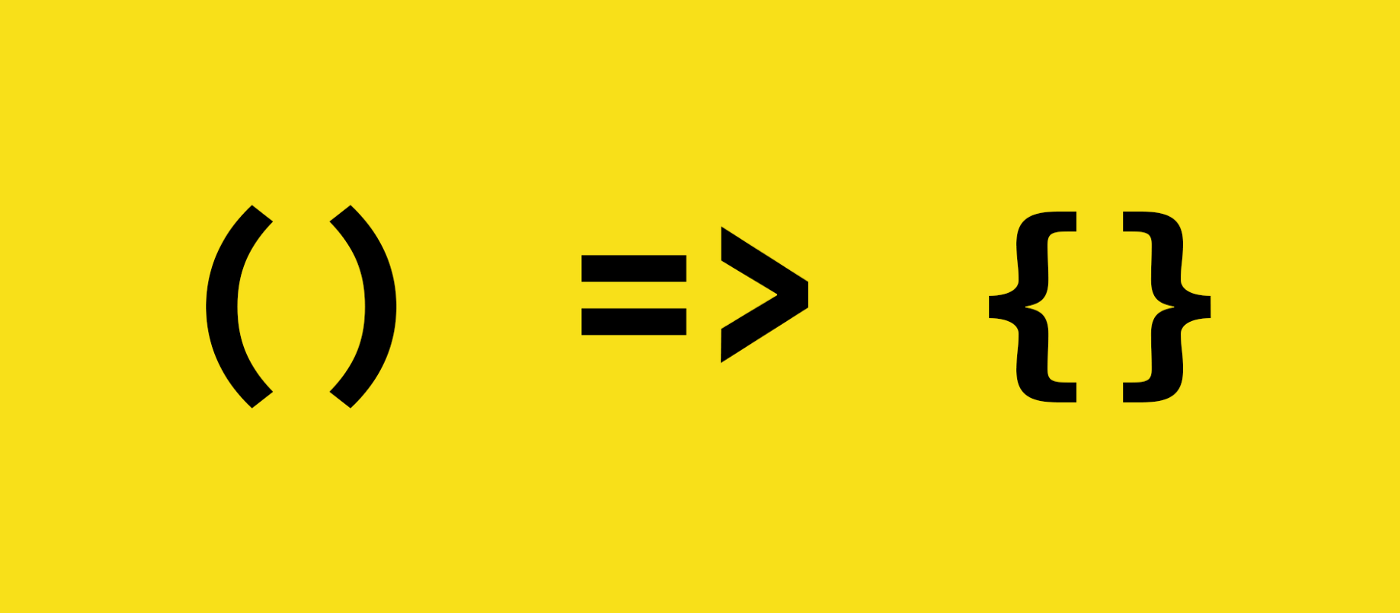
Difference Between Arrow Function and Regular Function
Arrow Function is introduced in ES6 version.Both regular and arrow function has similar working manner, but there are few Differences. let's talk about that.
Syntax: The expression of regular function and arrow function are different. You can do similar work with arrow with fewer lines of code compared the regular function. The curly brackets are not required for arrow function if only one expression is present.
Arguments Binding: Arrow functions do not have an arguments binding. However, they have access to the arguments object of the closest non-arrow parent function. Named and rest parameters are heavily relied upon to capture the arguments passed to arrow functions.
This keyword: Arrow funciton doesn't have own this-keyword. The value of this inside an arrow function returns the value of the closest non arrow parent funciton. On the other hand regular function has their own this keyword.
Regular functions created using function declarations or expressions are constructible and callable. Since regular functions are constructible, they can be called using the new keyword. However, the arrow functions are only callable and not constructible, i.e arrow functions can never be used as constructor functions. Hence, they can never be invoked with the new keyword.
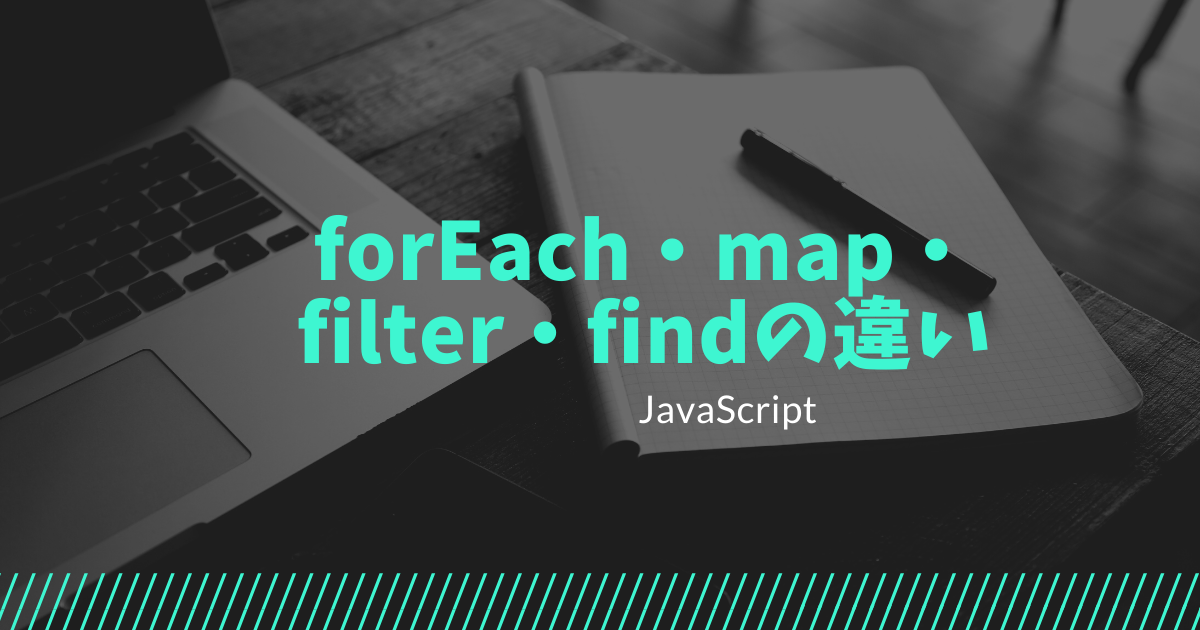
Difference Between Map, ForEach, Filter and Find
Map, ForEach, Filter and find are interesting method used for traversing an array.
Map: Map takes an array and run it against every elements of that array. But what makes it unique is it generate a new array based on the existing array.
ForEach: Foreach takes a callback function and run that callback function on each element of array one by one. Basically forEach works as a traditional for loop looping over the array and providing you array elements to do operations on them.
Filter: The main difference between forEach and filter is that forEach just loop over the array and executes the callback but filter executes the callback and check its return value. If the value is true element remains in the resulting array but if the return value is false the element will be removed for the resulting array.
Find: Find is same as the filter. Filter takes an array and return all the element if it matchs the condition. On the other hand find just return the first element which matches the condition.
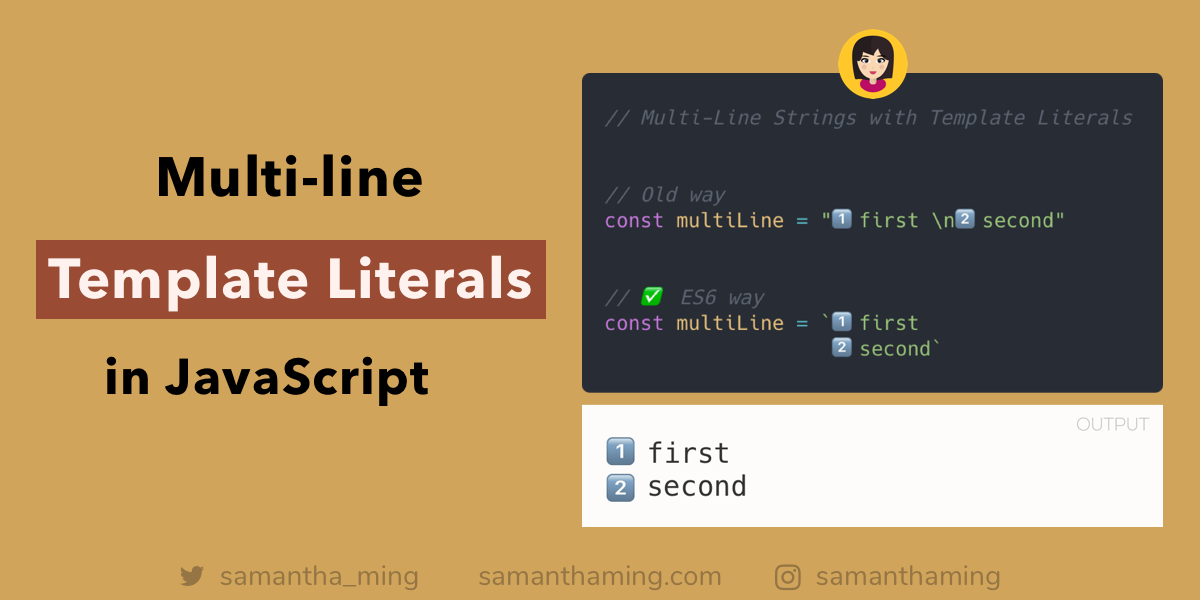
Why we should use template String
Template string comes to saves our valuable time. Before coming of template string we have to strugle a lot for creating multiline of string, dynamic string etc.
Template String or template literals helps us create multiline string without using any string concatenation or \n for print a new line. You can write multiline just inside the backtick(``). You don't have to use any + or \n sign.
Crete a dynamic string is very easy by using template string. We can set any dynamic value inside backtick(``) by using ${} sign. It saves a lot of time
So this is the reason we should use template string.